History
The initial development of C occurred at AT&T Bell Labs between 1969 and 1973; according to Ritchie, the most creative period occurred in 1972. It was named "C" because many of its
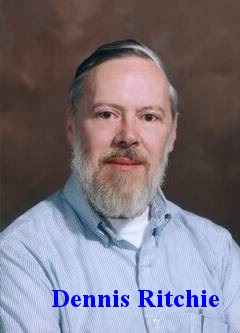
The origin of C is closely tied to the development of the Unix operating system, originally implemented in assembly language on a PDP-7 by Ritchie and Thompson, incorporating several ideas from colleagues. Eventually they decided to port the operating system to a PDP-11. B's lack of functionality to take advantage of some of the PDP-11's features, notably byte addressability, led to the development of an early version of the C programming language.
The original PDP-11 version of the Unix system was developed in assembly language. By 1973, with the addition of struct types, the
C language had become powerful enough that most of the Unix kernel was rewritten in C. This was one of the first operating system kernels implemented in a language other than assembly. (Earlier instances include the Multics system (written in PL/I), and MCP (Master Control Program) for the Burroughs B5000 written in ALGOL in 1961.)
What is c
C is a general-purpose computer programming language originally developed in 1972 by Dennis Ritchie at the Bell Telephone Laboratories to implement the Unix operating system.
Although C was designed for writing architecturally independent system software, it is also widely used for developing application software.
Worldwide, C is the first or second most popular language in terms of number of developer positions or publicly available code. It is widely used on many different software platforms, and there are few computer architectures for which a C compiler does not exist. C has greatly influenced many other popular programming languages, most notably C++, which originally began as an extension to C, and Java and C# which borrow C lexical conventions and operators.
Philosophy
C is an imperative (procedural) systems implementation language. It was designed to be compiled using a relatively straightforward compiler, to provide low-level access to memory, to provide language constructs that map efficiently to machine instructions, and to require minimal run-time support. C was therefore useful for many applications that had formerly been coded in assembly language.
Despite its low-level capabilities, the language was designed to encourage machine-independent programming. A standards-compliant and portably written C program can be compiled for a very wide variety of computer platforms and operating systems with little or no change to its source code, while approaching highest performance. The language has become available on a very wide range of platforms, from embedded microcontrollers to supercomputers.
Minimalism
C is designed to provide high-level abstracts for all the native features of a general-purpose CPU, while at the same time allowing modularization, structure, and code re-use. Features specific to a particular program's function (features that are not general to all platforms) are not included in the language or library definitions. However any such specific functions are implementable and accessible as external reusable libraries, in order to encourage module dissemination and re-use. C is somewhat strongly typed (emitting warnings or errors) but allows programmers to override types in the interests of flexibility, simplicity or performance; while being natural and well-defined in its interpretation of type overrides.
C's design is tied to its intended use as a portable systems implementation language. Consequently, it does not require run-time checks for conditions that would never occur in correct programs, it provides simple, direct access to any addressable object (for example, memory-mapped device control registers), and its source-code expressions can be translated in a straightforward manner to primitive machine operations in the executable code. Some early C compilers were comfortably implemented (as a few distinct passes communicating via intermediate files) on PDP-11 processors having only 16 address bits; however, C99 assumes a 512 KB minimum compilation platform.
Characteristics
Like most imperative languages in the ALGOL tradition, C has facilities for structured programming and allows lexical variable scope and recursion, while a static type system prevents many unintended operations. In C, all executable code is contained within functions. Function parameters are always passed by value. Pass-by-reference is achieved in C by explicitly passing pointer values. Heterogeneous aggregate data types (struct) allow related data elements to be combined and manipulated as a unit. C program source text is free-format, using the semicolon as a statement terminator (not a delimiter).
C also exhibits the following more specific characteristics:
* non-nestable function definitions
* variables may be hidden in nested blocks
* partially weak typing; for instance, characters can be used as integers
* low-level access to computer memory by converting machine addresses to typed pointers
* function and data pointers supporting ad hoc run-time polymorphism
* array indexing as a secondary notion, defined in terms of pointer arithmetic
* a preprocessor for macro definition, source code file inclusion, and conditional compilation
* complex functionality such as I/O, string manipulation, and mathematical functions consistently delegated to library routines
* A relatively small set of reserved keywords (originally 32, now 37 in C99)
* A lexical structure that resembles B more than ALGOL, for example
1.) { ... } rather than ALGOL's begin ... end
2.) the equal-sign is for assignment (copying), much like Fortran
3.) two consecutive equal-signs are to test for equality (compare to .EQ. in Fortran or the equal-sign in BASIC)
4.) && and || in place of ALGOL's and and or (these are semantically distinct from the bit-wise operators & and | because they will never evaluate the right operand if the result can be determined from the left alone (short-circuit evaluation)).
5.) a large number of compound operators, such as +=, ++, ......
Absent features
The relatively low-level nature of the language affords the programmer close control over what the computer does, while allowing special tailoring and aggressive optimization for a particular platform. This allows the code to run efficiently on very limited hardware, such as embedded systems.
C does not have some features that are available in some other programming languages:
* No assignment of arrays or strings (copying can be done via standard functions; assignment of objects having struct or union type is supported)
* No automatic garbage collection
* No requirement for bounds checking of arrays
* No operations on whole arrays
* No syntax for ranges, such as the A..B notation used in several languages
* No separate Boolean type: zero/nonzero is used instead
* No formal closures or functions as parameters (only function and variable pointers)
* No generators or coroutines; intra-thread control flow consists of nested function calls, except for the use of the longjmp or setcontext library functions
* No exception handling; standard library functions signify error conditions with the global errno variable and/or special return values
* Only rudimentary support for modular programming
* No compile-time polymorphism in the form of function or operator overloading
* Only rudimentary support for generic programming
* Very limited support for object-oriented programming with regard to polymorphism and inheritance
* Limited support for encapsulation
* No native support for multithreading and networking
* No standard libraries for computer graphics and several other application programming needs
A number of these features are available as extensions in some compilers, or can be supplied by third-party libraries, or can be simulated by adopting certain coding disciplines.
Uses
C's primary use is for "system programming", including implementing operating systems and embedded system applications, due to a combination of desirable characteristics such as code portability and efficiency, ability to access specific hardware addresses, ability to "pun" types to match externally imposed data access requirements, and low runtime demand on system resources.
One consequence of C's wide acceptance and efficiency is that C is used for other programs that are not directly used by end-users (compilers, libraries, interpreters, etc).
C is sometimes used as an intermediate language by implementations of other languages. This approach may be used for convenience (by using C as an intermediate language, it is not necessary to develop machine-specific code generators; ). Some compilers which use C this way are BitCC, Gambit, the Glasgow Haskell Compiler, Squeak, and valac.
Unfortunately, C was designed as a programming language, not as a compiler target language, and is thus less than ideal for use as an intermediate language. This has led to development of C-based intermediate languages such as C--.
C has also been widely used to implement end-user applications. Although as applications became larger, much of that development shifted to other languages.
Example program
The "hello, world" example which appeared in the first edition of K&R has become the model for an introductory program in most programming textbooks, regardless of programming language. The program prints "hello, world" to the standard output, which is usually a terminal or screen display. A standard-conforming "hello, world" program is
#include
int main(void)
{
printf("hello, world\n");
return 0;
}